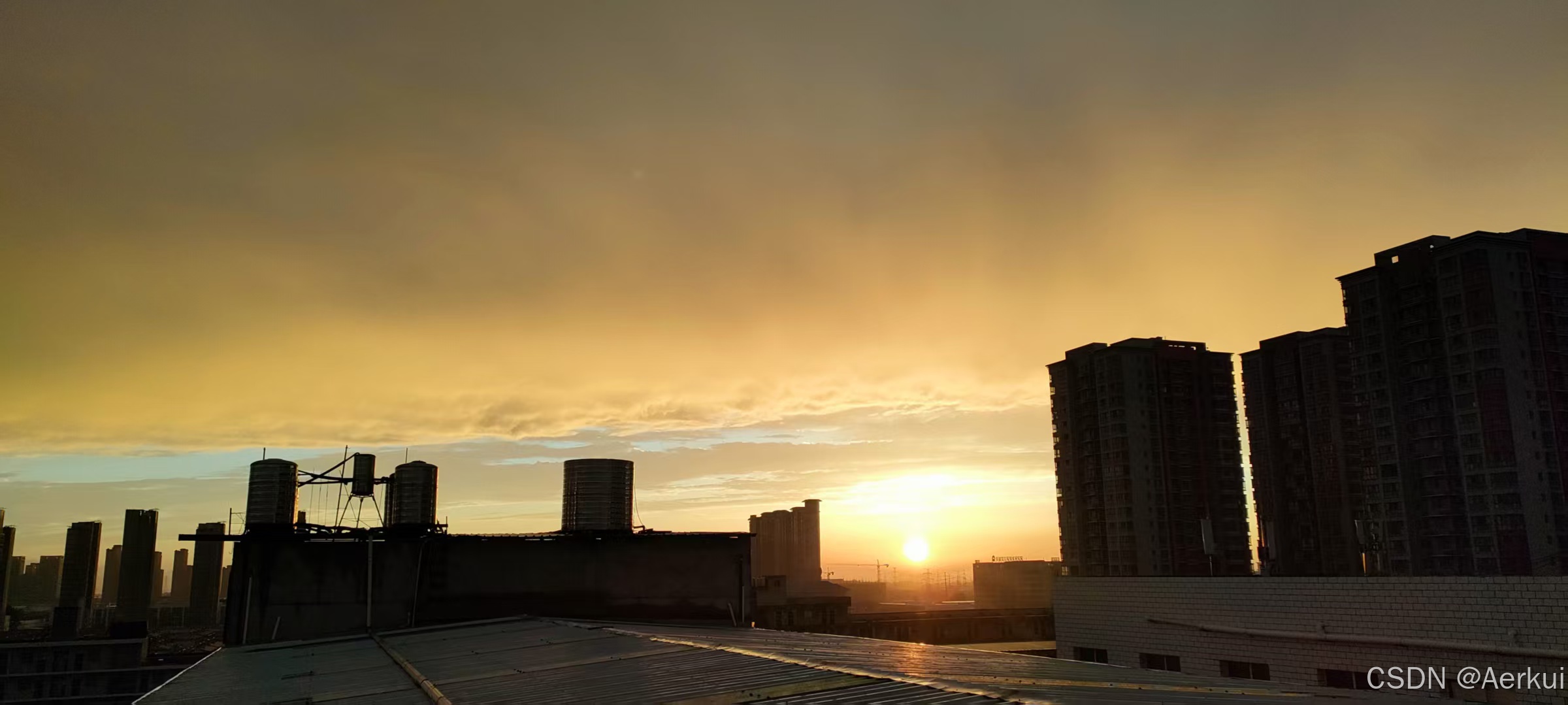
Python基础语法 - 判断语句
1. if语句
if 条件:
示例
age = 18
if age >= 18:print("您已成年")
2. if-else语句
if 条件:
else:
示例
age = 16
if age >= 18:print("您已成年")
else:print("您未成年")
3. if-elif-else语句
if 条件1:
elif 条件2:
else:
示例
score = 85
if score >= 90:print("优秀")
elif score >= 80:print("良好")
elif score >= 60:print("及格")
else:print("不及格")
4. 嵌套if语句
if 条件1:if 条件2:
示例
num = 15
if num > 0:if num % 2 == 0:print("正偶数")else:print("正奇数")
else:print("非正数")
5. 简写形式(三元运算符)
变量 = 值1 if 条件 else 值2
示例
age = 20
status = "成年" if age >= 18 else "未成年"
print(status)
6. 注意事项
- 冒号(
:
)不能省略 - 缩进必须一致(通常4个空格)
- 条件表达式可以是:
- 比较运算(
==
, !=
, >
, <
, >=
, <=
) - 逻辑运算(
and
, or
, not
) - 成员运算(
in
, not in
) - 布尔值(
True
, False
)
7. 实际应用示例
username = input("请输入用户名:")
password = input("请输入密码:")if username == "admin" and password == "123456":print("登录成功")
else:print("用户名或密码错误")