前言
本篇博客我们来看看C++11里发展了什么新的东西,跟C++98比起来有什么不同
💓 个人主页:小张同学zkf
⏩ 文章专栏:C++
若有问题 评论区见📝
🎉欢迎大家点赞👍收藏⭐文章
目录
1.C++11的发展历史
2.列表初始化
2.1C++98传统的{}
2.2C++11传统的{}
2.3C++11中的std::initializer_list
3.右值引用与移动语义
3.1左值与右值
3.2左值引用和右值引用
3.3引用延长生命周期
3.4左值和右值的参数匹配
3.5右值引用和移动语义的使用场景
3.5.1左值引用主要使用场景回顾
3.5.2移动构造和移动赋值
3.5.3右值引用和移动语义解决传值返回问题
3.6类型分类
3.7引用折叠
3.8完美转发
4.可变模版参数
4.1基本语法及原理
4.2包扩展
4.3empalce系列接口
5.新的类功能
5.1默认的移动构造和移动赋值
5.2defult和delete
6.STL中⼀些变化
7.lambda
7.1lambda表达式语法
7.2捕捉列表
7.3lambda的应用
7.4lambda的原理
8.包装器
8.1function
8.2bind
1.C++11的发展历史
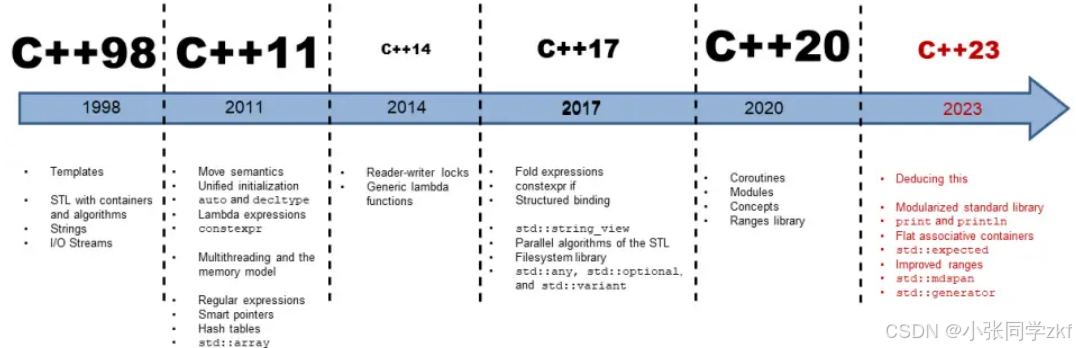
2.列表初始化
2.1C++98传统的{}
struct Point{int _x;int _y;};int main (){int array1[] = { 1 , 2 , 3 , 4 , 5 };int array2[ 5 ] = { 0 };Point p = { 1 , 2 };return 0 ;}
2.2C++11传统的{}
# include <iostream># include <vector>using namespace std;struct Point{int _x;int _y;};class Date{public :Date ( int year = 1 , int month = 1 , int day = 1 ):_year(year), _month(month), _day(day){cout << "Date(int year, int month, int day)" << endl;}Date ( const Date& d):_year(d._year), _month(d._month), _day(d._day){cout << "Date(const Date& d)" << endl;}private :int _year;int _month;int _day;};// ⼀切皆可⽤列表初始化,且可以不加 =int main (){// C++98 ⽀持的int a1[] = { 1 , 2 , 3 , 4 , 5 };int a2[ 5 ] = { 0 };Point p = { 1 , 2 };// C++11 ⽀持的// 内置类型⽀持int x1 = { 2 };// ⾃定义类型⽀持// 这⾥本质是⽤ { 2025, 1, 1} 构造⼀个 Date 临时对象// 临时对象再去拷⻉构造 d1 ,编译器优化后合⼆为⼀变成 { 2025, 1, 1} 直接构造初始化d1// 运⾏⼀下,我们可以验证上⾯的理论,发现是没调⽤拷⻉构造的Date d1 = { 2025 , 1 , 1 };// 这⾥ d2 引⽤的是 { 2024, 7, 25 } 构造的临时对象const Date& d2 = { 2024 , 7 , 25 };// 需要注意的是 C++98 ⽀持单参数时类型转换,也可以不⽤ {}Date d3 = { 2025 };Date d4 = 2025 ;// 可以省略掉 =Point p1 { 1 , 2 };int x2 { 2 };Date d6 { 2024 , 7 , 25 };const Date& d7 { 2024 , 7 , 25 };// 不⽀持,只有 {} 初始化,才能省略 =// Date d8 2025;vector<Date> v;v. push_back (d1);v. push_back ( Date ( 2025 , 1 , 1 ));// ⽐起有名对象和匿名对象传参,这⾥ {} 更有性价⽐v. push_back ({ 2025 , 1 , 1 });return 0 ;}
2.3C++11中的std::initializer_list
// STL 中的容器都增加了⼀个 initializer_list 的构造vector (initializer_list<value_type> il, const allocator_type& alloc =allocator_type ());list (initializer_list<value_type> il, const allocator_type& alloc =allocator_type ());map (initializer_list<value_type> il, const key_compare& comp =key_compare (), const allocator_type& alloc = allocator_type ());// ...template < class T >class vector {public :typedef T* iterator;vector (initializer_list<T> l){for ( auto e : l)push_back (e)}private :iterator _start = nullptr ;iterator _finish = nullptr ;iterator _endofstorage = nullptr ;};// 另外,容器的赋值也⽀持 initializer_list 的版本vector& operator = (initializer_list<value_type> il);map& operator = (initializer_list<value_type> il);
# include <iostream> # include <vector># include <string># include <map>using namespace std;int main (){std::initializer_list< int > mylist;mylist = { 10 , 20 , 30 };cout << sizeof (mylist) << endl;// 这⾥ begin 和 end 返回的值 initializer_list 对象中存的两个指针// 这两个指针的值跟 i 的地址跟接近,说明数组存在栈上int i = 0 ;cout << mylist. begin () << endl;cout << mylist. end () << endl;cout << &i << endl;// {} 列表中可以有任意多个值// 这两个写法语义上还是有差别的,第⼀个 v1 是直接构造,// 第⼆个 v2 是构造临时对象 + 临时对象拷⻉ v2+ 优化为直接构造vector< int > v1 ({ 1 , 2 , 3 , 4 , 5 });vector< int > v2 = { 1 , 2 , 3 , 4 , 5 };const vector< int >& v3 = { 1 , 2 , 3 , 4 , 5 };// 这⾥是 pair 对象的 {} 初始化和 map 的 initializer_list 构造结合到⼀起⽤了map<string, string> dict = { { "sort" , " 排序 " }, { "string" , " 字符串 " }};// initializer_list 版本的赋值⽀持v1 = { 10 , 20 , 30 , 40 , 50 };return 0 ;}
3.右值引用与移动语义
3.1左值与右值
# include <iostream>using namespace std;int main (){// 左值:可以取地址// 以下的 p 、 b 、 c 、 *p 、 s 、 s[0] 就是常⻅的左值int * p = new int ( 0 );int b = 1 ;const int c = b;*p = 10 ;string s ( "111111" );s[ 0 ] = 'x' ;cout << &c << endl;cout << ( void *)&s[ 0 ] << endl;// 右值:不能取地址double x = 1.1 , y = 2.2 ;// 以下⼏个 10 、 x + y 、 fmin(x, y) 、 string("11111") 都是常⻅的右值10 ;x + y;fmin (x, y);string ( "11111" );//cout << &10 << endl;//cout << &(x+y) << endl;//cout << &(fmin(x, y)) << endl;//cout << &string("11111") << endl;return 0 ;}
3.2左值引用和右值引用
template < class _Ty >remove_reference_t <_Ty>&& move (_Ty&& _Arg){ // forward _Arg as movablereturn static_cast < remove_reference_t <_Ty>&&>(_Arg);}# include <iostream>using namespace std;int main (){// 左值:可以取地址// 以下的 p 、 b 、 c 、 *p 、 s 、 s[0] 就是常⻅的左值int * p = new int ( 0 );int b = 1 ;const int c = b;*p = 10 ;string s ( "111111" );s[ 0 ] = 'x' ;double x = 1.1 , y = 2.2 ;// 左值引⽤给左值取别名int & r1 = b;int *& r2 = p;int & r3 = *p;string& r4 = s;char & r5 = s[ 0 ];// 右值引⽤给右值取别名int && rr1 = 10 ;double && rr2 = x + y;double && rr3 = fmin (x, y);string&& rr4 = string ( "11111" );// 左值引⽤不能直接引⽤右值,但是 const 左值引⽤可以引⽤右值const int & rx1 = 10 ;const double & rx2 = x + y;const double & rx3 = fmin (x, y);const string& rx4 = string ( "11111" );// 右值引⽤不能直接引⽤左值,但是右值引⽤可以引⽤ move( 左值 )int && rrx1 = move (b);int *&& rrx2 = move (p);int && rrx3 = move (*p);string&& rrx4 = move (s);string&& rrx5 = (string&&)s;// b 、 r1 、 rr1 都是变量表达式,都是左值cout << &b << endl;cout << &r1 << endl;cout << &rr1 << endl;// 这⾥要注意的是, rr1 的属性是左值,所以不能再被右值引⽤绑定,除⾮ move ⼀下int & r6 = r1;// int&& rrx6 = rr1;int && rrx6 = move (rr1);return 0 ;}
3.3引用延长生命周期
int main (){std::string s1 = "Test" ;// std::string&& r1 = s1; // 错误:不能绑定到左值const std::string& r2 = s1 + s1; // OK :到 const 的左值引⽤延⻓⽣存期// r2 += "Test"; // 错误:不能通过到 const 的引⽤修改std::string&& r3 = s1 + s1; // OK :右值引⽤延⻓⽣存期 r3 += "Test" ; // OK :能通过到⾮ const 的引⽤修改std::cout << r3 << '\n' ;return 0 ;}
3.4左值和右值的参数匹配
# include <iostream>using namespace std;void f ( int & x){std::cout << " 左值引⽤重载 f(" << x << ")\n" ;}void f ( const int & x){std::cout << " 到 const 的左值引⽤重载 f(" << x << ")\n" ;}void f ( int && x){std::cout << " 右值引⽤重载 f(" << x << ")\n" ;}int main (){int i = 1 ;const int ci = 2 ;f (i); // 调⽤ f(int&)f (ci); // 调⽤ f(const int&)f ( 3 ); // 调⽤ f(int&&) ,如果没有 f(int&&) 重载则会调⽤ f(const int&)f (std:: move (i)); // 调⽤ f(int&&)// 右值引⽤变量在⽤于表达式时是左值int && x = 1 ;f (x); // 调⽤ f(int& x)f (std:: move (x)); // 调⽤ f(int&& x)return 0 ;}
3.5右值引用和移动语义的使用场景
3.5.1左值引用主要使用场景回顾
class Solution {public :// 传值返回需要拷⻉string addStrings (string num1, string num2) {string str;int end1 = num1. size () -1 , end2 = num2. size () -1 ;// 进位int next = 0 ;while (end1 >= 0 || end2 >= 0 ){int val1 = end1 >= 0 ? num1[end1--]- '0' : 0 ;int val2 = end2 >= 0 ? num2[end2--]- '0' : 0 ;int ret = val1 + val2+next;next = ret / 10 ;ret = ret % 10 ;str += ( '0' +ret);}if (next == 1 )str += '1' ;reverse (str. begin (), str. end ());return str;}};class Solution {public :// 这⾥的传值返回拷⻉代价就太⼤了vector<vector< int >> generate ( int numRows) {vector<vector< int >> vv (numRows);for ( int i = 0 ; i < numRows; ++i){vv[i]. resize (i+ 1 , 1 );}for ( int i = 2 ; i < numRows; ++i){for ( int j = 1 ; j < i; ++j){vv[i][j] = vv[i -1 ][j] + vv[i -1 ][j -1 ];}}return vv;}};
3.5.2移动构造和移动赋值
1# define _CRT_SECURE_NO_WARNINGS 12# include <iostream>3# include <assert.h>4# include <string.h>5# include <algorithm>6using namespace std;78namespace bit9 {10 class string11 {12 public :13 typedef char * iterator;14 typedef const char * const_iterator;1516 iterator begin ()17 {18 return _str;19 }20 iterator end ()21 {22 return _str + _size;23 }2425 const_iterator begin () const26 {27 return _str;28 }2930 const_iterator end () const31 {32 return _str + _size;33 }3435 string ( const char * str = "" )36 :_size( strlen (str))37 , _capacity(_size)38 {39 cout << "string(char* str)- 构造 " << endl;40 _str = new char [_capacity + 1 ];41 strcpy (_str, str);42 }4344 void swap (string& s)45 {46 :: swap (_str, s._str);47 :: swap (_size, s._size);48 :: swap (_capacity, s._capacity);49 }5051 string ( const string& s)52 :_str( nullptr )53 {54 cout << "string(const string& s) -- 拷⻉构造 " << endl;5556 reserve (s._capacity);57 for ( auto ch : s)58 {59 push_back (ch);60 }61 }6263 // 移动构造64 string (string&& s)65 {66 cout << "string(string&& s) -- 移动构造 " << endl;67 swap (s);68 }6970 string& operator =( const string& s)71 {72 cout << "string& operator=(const string& s) -- 拷⻉赋值 " <<endl;73 if ( this != &s)74 {75 _str[ 0 ] = '\0' ;76 _size = 0 ;7778 reserve (s._capacity);79 for ( auto ch : s)80 {81 push_back (ch);82 }83 }8485 return * this ;86 }8788 // 移动赋值89 string& operator =(string&& s)90 {91 cout << "string& operator=(string&& s) -- 移动赋值 " << endl;92 swap (s);93 return * this ;94 }9596 ~ string ()97 {98 cout << "~string() -- 析构 " << endl;99 delete [] _str;100 _str = nullptr ;101 }102103 char & operator []( size_t pos)104 {105 assert (pos < _size);106 return _str[pos];107 }108109 void reserve ( size_t n)110 {111 if (n > _capacity)112 {113 char * tmp = new char [n + 1 ];114 if (_str)115 {116 strcpy (tmp, _str);117 delete [] _str;118 }119 _str = tmp;120 _capacity = n;121 }122 }123124 void push_back ( char ch)125 {126 if (_size >= _capacity)127 {128 size_t newcapacity = _capacity == 0 ? 4 : _capacity * 2 ;129 reserve (newcapacity);130 }131132 _str[_size] = ch;133 ++_size;134 _str[_size] = '\0' ;135 }136137 string& operator +=( char ch)138 {139 push_back (ch);140 return * this ;141 }142143 const char * c_str () const144 {145 return _str;146 }147 148 size_t size () const149 {150 return _size;151 }152 private :153 char * _str = nullptr ;154 size_t _size = 0 ;155 size_t _capacity = 0 ;156 };157 }158159 int main ()160 {161 bit::string s1 ( "xxxxx" );162 // 拷⻉构造163 bit::string s2 = s1;164 // 构造 + 移动构造,优化后直接构造165 bit::string s3 = bit:: string ( "yyyyy" );166 // 移动构造167 bit::string s4 = move (s1);168 cout << "******************************" << endl;169170 return 0 ;171 }
3.5.3右值引用和移动语义解决传值返回问题
namespace bit{string addStrings (string num1, string num2){string str;int end1 = num1. size () - 1 , end2 = num2. size () - 1 ;int next = 0 ;while (end1 >= 0 || end2 >= 0 ){int val1 = end1 >= 0 ? num1[end1--] - '0' : 0 ;int val2 = end2 >= 0 ? num2[end2--] - '0' : 0 ;int ret = val1 + val2 + next;next = ret / 10 ;ret = ret % 10 ;str += ( '0' + ret);} if (next == 1 )str += '1' ;reverse (str. begin (), str. end ());cout << "******************************" << endl;return str;}}// 场景 1int main (){bit::string ret = bit:: addStrings ( "11111" , "2222" );cout << ret. c_str () << endl;return 0 ;}// 场景 2int main (){bit::string ret;ret = bit:: addStrings ( "11111" , "2222" );cout << ret. c_str () << endl;return 0 ;}
3.6类型分类
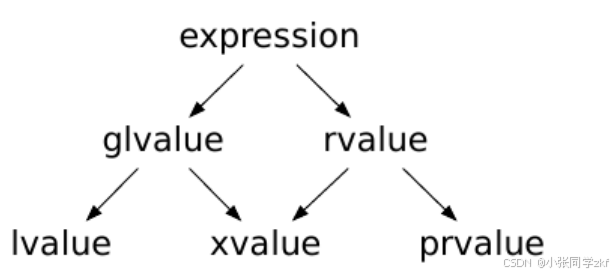
3.7引用折叠
// 由于引⽤折叠限定, f1 实例化以后总是⼀个左值引⽤template < class T>void f1 (T& x){}// 由于引⽤折叠限定, f2 实例化后可以是左值引⽤,也可以是右值引⽤template < class T>void f2 (T&& x){}int main (){typedef int & lref;typedef int && rref;int n = 0 ;lref& r1 = n; // r1 的类型是 int&lref&& r2 = n; // r2 的类型是 int&rref& r3 = n; // r3 的类型是 int&rref&& r4 = 1 ; // r4 的类型是 int&&// 没有折叠 -> 实例化为 void f1(int& x)f1 < int >(n);f1 < int >( 0 ); // 报错// 折叠 -> 实例化为 void f1(int& x)f1 < int &>(n);f1 < int &>( 0 ); // 报错// 折叠 -> 实例化为 void f1(int& x)f1 < int &&>(n);f1 < int &&>( 0 ); // 报错// 折叠 -> 实例化为 void f1(const int& x)f1 < const int &>(n);f1 < const int &>( 0 );// 折叠 -> 实例化为 void f1(const int& x)f1 < const int &&>(n);f1 < const int &&>( 0 );// 没有折叠 -> 实例化为 void f2(int&& x)f2 < int >(n); // 报错f2 < int >( 0 );// 折叠 -> 实例化为 void f2(int& x)f2 < int &>(n);f2 < int &>( 0 ); // 报错// 折叠 -> 实例化为 void f2(int&& x)f2 < int &&>(n); // 报错f2 < int &&>( 0 );return 0 ;}
1 template < class T>2 void Function (T&& t)3 {4 int a = 0 ;5 T x = a;6 //x++;7 cout << &a << endl;8 cout << &x << endl << endl;9 }1011 int main ()12 {13 // 10 是右值,推导出 T 为 int ,模板实例化为 void Function(int&& t)14 Function ( 10 ); // 右值1516 int a;17 // a 是左值,推导出 T 为 int& ,引⽤折叠,模板实例化为 void Function(int& t)18 Function (a); // 左值1920 // std::move(a) 是右值,推导出 T 为 int ,模板实例化为 void Function(int&& t)21 Function (std:: move (a)); // 右值2223 const int b = 8 ;24 // a 是左值,推导出 T 为 const int& ,引⽤折叠,模板实例化为 void Function(const int&t)25 // 所以 Function 内部会编译报错, x 不能 ++26 Function (b); // const 左值27// std::move(b) 右值,推导出 T 为 const int ,模板实例化为 void Function(const int&&t)// 所以 Function 内部会编译报错, x 不能 ++Function (std:: move (b)); // const 右值return 0 ;}
3.8完美转发
template < class _Ty >_Ty&& forward ( remove_reference_t <_Ty>& _Arg) noexcept{ // forward an lvalue as either an lvalue or an rvaluereturn static_cast <_Ty&&>(_Arg);}void Fun ( int & x) { cout << " 左值引⽤ " << endl; }void Fun ( const int & x) { cout << "const 左值引⽤ " << endl; }void Fun ( int && x) { cout << " 右值引⽤ " << endl; }void Fun ( const int && x) { cout << "const 右值引⽤ " << endl; }template < class T>void Function (T&& t){Fun (t);//Fun(forward<T>(t));}int main (){// 10 是右值,推导出 T 为 int ,模板实例化为 void Function(int&& t)Function ( 10 ); // 右值int a;// a 是左值,推导出 T 为 int& ,引⽤折叠,模板实例化为 void Function(int& t)Function (a); // 左值// std::move(a) 是右值,推导出 T 为 int ,模板实例化为 void Function(int&& t)Function (std:: move (a)); // 右值const int b = 8 ;// a 是左值,推导出 T 为 const int& ,引⽤折叠,模板实例化为 void Function(const int&t)Function (b); // const 左值// std::move(b) 右值,推导出 T 为 const int ,模板实例化为 void Function(const int&&t)Function (std:: move (b)); // const 右值return 0 ;}
4.可变模版参数
4.1基本语法及原理
template < class ...Args>void Print (Args&&... args){cout << sizeof ...(args) << endl;}int main (){double x = 2.2 ;Print (); // 包⾥有 0 个参数Print ( 1 ); // 包⾥有 1 个参数Print ( 1 , string ( "xxxxx" )); // 包⾥有 2 个参数Print ( 1.1 , string ( "xxxxx" ), x); // 包⾥有 3 个参数return 0 ;}// 原理 1 :编译本质这⾥会结合引⽤折叠规则实例化出以下四个函数void Print ();void Print ( int && arg1);void Print ( int && arg1, string&& arg2);void Print ( double && arg1, string&& arg2, double & arg3);// 原理 2 :更本质去看没有可变参数模板,我们实现出这样的多个函数模板才能⽀持// 这⾥的功能,有了可变参数模板,我们进⼀步被解放,他是类型泛化基础// 上叠加数量变化,让我们泛型编程更灵活。void Print ();template < class T1 >void Print (T1&& arg1);template < class T1 , class T2 >void Print (T1&& arg1, T2&& arg2);template < class T1 , class T2 , class T3 >void Print (T1&& arg1, T2&& arg2, T3&& arg3);// ...
4.2包扩展
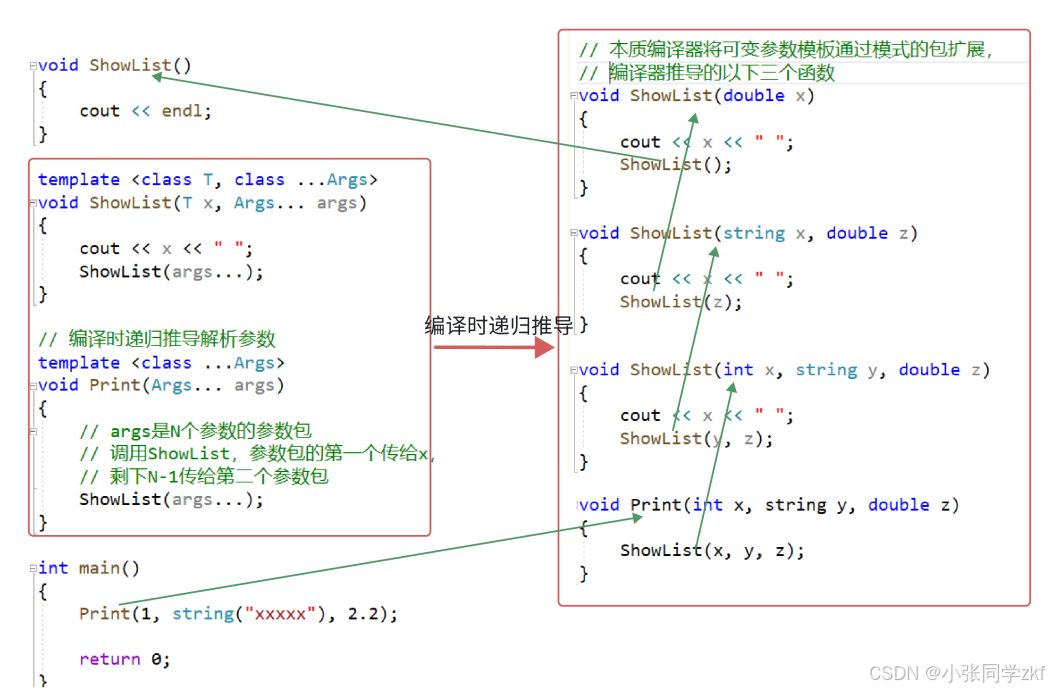
1// 可变模板参数2// 参数类型可变3// 参数个数可变4// 打印参数包内容5//template <class ...Args>6//void Print(Args... args)7//{8// // 可变参数模板编译时解析9// // 下⾯是运⾏获取和解析,所以不⽀持这样⽤10// cout << sizeof...(args) << endl;11// for (size_t i = 0; i < sizeof...(args); i++)12// {13// cout << args[i] << " ";14// }15 // cout << endl;16 //}1718 void ShowList ()19 {20 // 编译器时递归的终⽌条件,参数包是 0 个时,直接匹配这个函数21 cout << endl;22 }2324 template < class T , class ...Args>25 void ShowList (T x, Args... args)26 {27 cout << x << " " ;28 // args 是 N 个参数的参数包29 // 调⽤ ShowList ,参数包的第⼀个传给 x ,剩下 N-1 传给第⼆个参数包30 ShowList (args...);31 }3233 // 编译时递归推导解析参数34 template < class ...Args>35 void Print (Args... args)36 {37 ShowList (args...);38 }3940 int main ()41 {42 Print ();43 Print ( 1 );44 Print ( 1 , string ( "xxxxx" ));45 Print ( 1 , string ( "xxxxx" ), 2.2 );4647 return 0 ;48 }4950 //template <class T, class ...Args>51 //void ShowList(T x, Args... args)52 //{53 // cout << x << " ";54 // Print(args...);55 //}5657 // Print(1, string("xxxxx"), 2.2); 调⽤时58 // 本质编译器将可变参数模板通过模式的包扩展,编译器推导的以下三个重载函数函数59 //void ShowList(double x)60 //{61 // cout << x << " "; 62 // ShowList();63 //}64 //65 //void ShowList(string x, double z)66 //{67 // cout << x << " ";68 // ShowList(z);69 //}70 //71 //void ShowList(int x, string y, double z)72 //{73 // cout << x << " ";74 // ShowList(y, z);75 //}7677 //void Print(int x, string y, double z)78 //{79 // ShowList(x, y, z);80 //}
1 template < class T >2 const T& GetArg ( const T& x)3 {4 cout << x << " " ;5 return x;6 }78 template < class ...Args>9 void Arguments (Args... args)10 {}1112 template < class ...Args>13 void Print (Args... args)14 {15 // 注意 GetArg 必须返回或者到的对象,这样才能组成参数包给 Arguments16 Arguments ( GetArg (args)...);17 }1819 // 本质可以理解为编译器编译时,包的扩展模式20 // 将上⾯的函数模板扩展实例化为下⾯的函数21 // 是不是很抽象, C++11 以后,只能说委员会的⼤佬设计语法思维跳跃得太厉害22 //void Print(int x, string y, double z)23 //{24 // Arguments(GetArg(x), GetArg(y), GetArg(z));25 //}int main (){Print ( 1 , string ( "xxxxx" ), 2.2 );return 0 ;}
4.3empalce系列接口
1# include <list>2// emplace_back 总体⽽⾔是更⾼效,推荐以后使⽤ emplace 系列替代 insert 和 push 系列3int main ()4{5list<bit::string> lt;6// 传左值,跟 push_back ⼀样,⾛拷⻉构造7bit::string s1 ( "111111111111" );8lt. emplace_back (s1);9cout << "*********************************" << endl;10// 右值,跟 push_back ⼀样,⾛移动构造11lt. emplace_back ( move (s1));12cout << "*********************************" << endl;13// 直接把构造 string 参数包往下传,直接⽤ string 参数包构造 string14// 这⾥达到的效果是 push_back 做不到的15lt. emplace_back ( "111111111111" );19 cout << "*********************************" << endl;2021 list<pair<bit::string, int >> lt1;22 // 跟 push_back ⼀样23 // 构造 pair + 拷⻉ / 移动构造 pair 到 list 的节点中 data 上24 pair<bit::string, int > kv ( " 苹果 " , 1 );25 lt1. emplace_back (kv);26 cout << "*********************************" << endl;2728 // 跟 push_back ⼀样29 lt1. emplace_back ( move (kv));30 cout << "*********************************" << endl;313233 // 直接把构造 pair 参数包往下传,直接⽤ pair 参数包构造 pair34 // 这⾥达到的效果是 push_back 做不到的35 lt1. emplace_back ( " 苹果 " , 1 );36 cout << "*********************************" << endl;3738 return 0 ;39 }
1 // List.h2 namespace bit3 {4 template < class T >5 struct ListNode6 {7 ListNode<T>* _next;8 ListNode<T>* _prev;910 T _data;1112 ListNode (T&& data)13 :_next( nullptr )14 , _prev( nullptr )15 , _data( move (data))16 {}1718 template < class ... Args>19 ListNode (Args&&... args)20 : _next( nullptr )21 , _prev( nullptr )22 , _data(std::forward<Args>(args)...)23 {} 24 };2526 template < class T , class Ref , class Ptr >27 struct ListIterator28 {29 typedef ListNode<T> Node;30 typedef ListIterator<T, Ref, Ptr> Self;31 Node* _node;3233 ListIterator (Node* node)34 :_node(node)35 {}3637 // ++it;38 Self& operator ++()39 {40 _node = _node->_next;41 return * this ;42 }4344 Self& operator --()45 {46 _node = _node->_prev;47 return * this ;48 }4950 Ref operator *()51 {52 return _node->_data;53 }5455 bool operator !=( const Self& it)56 {57 return _node != it._node;58 }59 };6061 template < class T >62 class list63 {64 typedef ListNode<T> Node;65 public :66 typedef ListIterator<T, T&, T*> iterator;67 typedef ListIterator<T, const T&, const T*> const_iterator;6869 iterator begin ()70 {71 return iterator (_head->_next);72 }7374 iterator end ()75 {76 return iterator (_head);77 }7879 void empty_init ()80 {81 _head = new Node ();82 _head->_next = _head;83 _head->_prev = _head;84 }8586 list ()87 {88 empty_init ();89 }9091 void push_back ( const T& x)92 {93 insert ( end (), x);94 }9596 void push_back (T&& x)97 {98 insert ( end (), move (x));99 }100101 iterator insert (iterator pos, const T& x)102 {103 Node* cur = pos._node;104 Node* newnode = new Node (x);105 Node* prev = cur->_prev;106107 // prev newnode cur108 prev->_next = newnode;109 newnode->_prev = prev;110 newnode->_next = cur;111 cur->_prev = newnode;112113 return iterator (newnode);114 }115116 iterator insert (iterator pos, T&& x)117 { 118 Node* cur = pos._node;119 Node* newnode = new Node ( move (x));120 Node* prev = cur->_prev;121122 // prev newnode cur123 prev->_next = newnode;124 newnode->_prev = prev;125 newnode->_next = cur;126 cur->_prev = newnode;127128 return iterator (newnode);129 }130131 template < class ... Args>132 void emplace_back (Args&&... args)133 {134 insert ( end (), std::forward<Args>(args)...);135 }136137 // 原理:本质编译器根据可变参数模板⽣成对应参数的函数138 /*void emplace_back(string& s)139 {140 insert(end(), std::forward<string>(s));141 }142143 void emplace_back(string&& s)144 {145 insert(end(), std::forward<string>(s));146 }147148 void emplace_back(const char* s)149 {150 insert(end(), std::forward<const char*>(s));151 }152 */153154 template < class ... Args>155 iterator insert (iterator pos, Args&&... args)156 {157 Node* cur = pos._node;158 Node* newnode = new Node (std::forward<Args>(args)...);159 Node* prev = cur->_prev;160161 // prev newnode cur162 prev->_next = newnode;163 newnode->_prev = prev;164 newnode->_next = cur; 165 cur->_prev = newnode;166167 return iterator (newnode);168 }169 private :170 Node* _head;171 };172 }173174 // Test.cpp175 # include "List.h"176177 // emplace_back 总体⽽⾔是更⾼效,推荐以后使⽤ emplace 系列替代 insert 和 push 系列178 int main ()179 {180 bit::list<bit::string> lt;181 // 传左值,跟 push_back ⼀样,⾛拷⻉构造182 bit::string s1 ( "111111111111" );183 lt. emplace_back (s1);184 cout << "*********************************" << endl;185186 // 右值,跟 push_back ⼀样,⾛移动构造187 lt. emplace_back ( move (s1));188 cout << "*********************************" << endl;189190 // 直接把构造 string 参数包往下传,直接⽤ string 参数包构造 string191 // 这⾥达到的效果是 push_back 做不到的192 lt. emplace_back ( "111111111111" );193 cout << "*********************************" << endl;194195 bit::list<pair<bit::string, int >> lt1;196 // 跟 push_back ⼀样197 // 构造 pair + 拷⻉ / 移动构造 pair 到 list 的节点中 data 上198 pair<bit::string, int > kv ( " 苹果 " , 1 );199 lt1. emplace_back (kv);200 cout << "*********************************" << endl;201202 // 跟 push_back ⼀样203 lt1. emplace_back ( move (kv));204 cout << "*********************************" << endl;205206207 // 直接把构造 pair 参数包往下传,直接⽤ pair 参数包构造 pair208 // 这⾥达到的效果是 push_back 做不到的209 lt1. emplace_back ( " 苹果 " , 1 );210 cout << "*********************************" << endl;211 return 0 ;}212213
5.新的类功能
5.1默认的移动构造和移动赋值
class Person{public :Person ( const char * name = "" , int age = 0 ):_name(name), _age(age){}/*Person(const Person& p):_name(p._name),_age(p._age){}*//*Person& operator=(const Person& p){if(this != &p){_name = p._name;_age = p._age;}return *this;}*//*~Person(){}*/private :bit::string _name;int _age;};int main (){Person s1;Person s2 = s1;Person s3 = std:: move (s1);Person s4;s4 = std:: move (s2);return 0 ;}
5.2defult和delete
class Person{public :Person ( const char * name = "" , int age = 0 ):_name(name), _age(age){}Person ( const Person& p):_name(p._name),_age(p._age){}Person (Person&& p) = default ;//Person(const Person& p) = delete;private :bit::string _name;int _age;};int main (){Person s1;Person s2 = s1;Person s3 = std:: move (s1);return 0 ;}
6.STL中⼀些变化
7.lambda
7.1lambda表达式语法
int main (){// ⼀个简单的 lambda 表达式auto add1 = []( int x, int y)-> int { return x + y; };cout << add1 ( 1 , 2 ) << endl;// 1 、捕捉为空也不能省略// 2 、参数为空可以省略// 3 、返回值可以省略,可以通过返回对象⾃动推导// 4 、函数题不能省略auto func1 = []{cout << "hello bit" << endl;return 0 ;};func1 ();int a = 0 , b = 1 ;auto swap1 = []( int & x, int & y){int tmp = x;x = y;y = tmp;};swap1 (a, b);cout << a << ":" << b << endl;return 0 ;}
7.2捕捉列表
int x = 0 ;// 捕捉列表必须为空,因为全局变量不⽤捕捉就可以⽤,没有可被捕捉的变量auto func1 = [](){x++;};int main (){// 只能⽤当前 lambda 局部域和捕捉的对象和全局对象int a = 0 , b = 1 , c = 2 , d = 3 ;auto func1 = [a, &b]{// 值捕捉的变量不能修改,引⽤捕捉的变量可以修改//a++;b++;int ret = a + b;return ret;};cout << func1 () << endl;// 隐式值捕捉// ⽤了哪些变量就捕捉哪些变量auto func2 = [=]{int ret = a + b + c;return ret;};cout << func2 () << endl;// 隐式引⽤捕捉// ⽤了哪些变量就捕捉哪些变量auto func3 = [&]{a++;c++;d++;};func3 ();cout << a << " " << b << " " << c << " " << d <<endl;// 混合捕捉 1auto func4 = [&, a, b]{//a++;//b++;c++;d++;return a + b + c + d;};func4 ();cout << a << " " << b << " " << c << " " << d << endl;// 混合捕捉 1auto func5 = [=, &a, &b]{a++;b++;/*c++;d++;*/return a + b + c + d;};func5 ();cout << a << " " << b << " " << c << " " << d << endl;// 局部的静态和全局变量不能捕捉,也不需要捕捉static int m = 0 ;auto func6 = []{int ret = x + m;return ret;};// 传值捕捉本质是⼀种拷⻉ , 并且被 const 修饰了// mutable 相当于去掉 const 属性,可以修改了// 但是修改了不会影响外⾯被捕捉的值,因为是⼀种拷⻉auto func7 = [=]() mutable{a++;b++;c++; d++;return a + b + c + d;};cout << func7 () << endl;cout << a << " " << b << " " << c << " " << d << endl;return 0 ;}
7.3lambda的应用
struct Goods{string _name; // 名字double _price; // 价格int _evaluate; // 评价// ...Goods ( const char * str, double price, int evaluate):_name(str), _price(price), _evaluate(evaluate){}};struct ComparePriceLess{bool operator ()( const Goods& gl, const Goods& gr){return gl._price < gr._price;}};struct ComparePriceGreater{bool operator ()( const Goods& gl, const Goods& gr){return gl._price > gr._price;}};int main (){vector<Goods> v = { { " 苹果 " , 2.1 , 5 }, { " ⾹蕉 " , 3 , 4 }, { " 橙⼦ " , 2.2 , 3}, { " 菠萝 " , 1.5 , 4 } };// 类似这样的场景,我们实现仿函数对象或者函数指针⽀持商品中// 不同项的⽐较,相对还是⽐较⿇烦的,那么这⾥ lambda 就很好⽤了sort (v. begin (), v. end (), ComparePriceLess ());sort (v. begin (), v. end (), ComparePriceGreater ());sort (v. begin (), v. end (), []( const Goods& g1, const Goods& g2) {return g1._price < g2._price;});sort (v. begin (), v. end (), []( const Goods& g1, const Goods& g2) {return g1._price > g2._price;});sort (v. begin (), v. end (), []( const Goods& g1, const Goods& g2) {return g1._evaluate < g2._evaluate;});sort (v. begin (), v. end (), []( const Goods& g1, const Goods& g2) {return g1._evaluate > g2._evaluate;});return 0 ;}
7.4lambda的原理
1 class Rate2 {3 public :4 Rate ( double rate)5 : _rate(rate)6 {}78 double operator ()( double money, int year)9 {10 return money * _rate * year;11 }1213 private :14 double _rate;15 };1617 int main ()18 {19 double rate = 0.49 ;2021 // lambda22 auto r2 = [rate]( double money, int year) {23 return money * rate * year;24 };2526 // 函数对象27 Rate r1 (rate);28 r1 ( 10000 , 2 );2930 r2 ( 10000 , 2 );3132 auto func1 = [] {33 cout << "hello world" << endl;34 };35 func1 ();3637 return 0 ;38 }39
1 // lambda2 auto r2 = [rate]( double money, int year) {3 return money * rate * year;4 };5 // 捕捉列表的 rate ,可以看到作为 lambda_1 类构造函数的参数传递了,这样要拿去初始化成员变量 6 // 下⾯ operator() 中才能使⽤7 00 D8295C lea eax,[rate]8 00 D8295F push eax9 00 D82960 lea ecx,[r2]10 00 D82963 call `main ' ::` 2' ::<lambda_1>::<lambda_1> ( 0 D81F80h)1112 // 函数对象13 Rate r1 (rate);14 00 D82968 sub esp, 815 00 D8296B movsd xmm0,mmword ptr [rate]16 00 D82970 movsd mmword ptr [esp],xmm017 00 D82975 lea ecx,[r1]18 00 D82978 call Rate::Rate ( 0 D81438h)19 r1 ( 10000 , 2 );20 00 D8297D push 221 00 D8297F sub esp, 822 00 D82982 movsd xmm0,mmword ptr [__real@ 40 c3880000000000 ( 0 D89B50h)]23 00 D8298A movsd mmword ptr [esp],xmm024 00 D8298F lea ecx,[r1]25 00 D82992 call Rate::operator () ( 0 D81212h)2627 // 汇编层可以看到 r2 lambda 对象调⽤本质还是调⽤ operator() ,类型是 lambda_1, 这个类型名28 // 的规则是编译器⾃⼰定制的,保证不同的 lambda 不冲突29 r2 ( 10000 , 2 );30 00 D82999 push 231 00 D8299B sub esp, 832 00 D8299E movsd xmm0,mmword ptr [__real@ 40 c3880000000000 ( 0 D89B50h)]33 00 D829A6 movsd mmword ptr [esp],xmm034 00 D829AB lea ecx,[r2]35 00 D829AE call `main ' ::` 2' ::<lambda_1>:: operator () ( 0 D824C0h)
8.包装器
8.1function
1 template < class T >2 class function ; // undefined34 template < class Ret , class ... Args>5 class function < Ret (Args...)>;
# include <functional>int f ( int a, int b){return a + b;}struct Functor{public :int operator () ( int a, int b){return a + b;}};class Plus{public :Plus ( int n = 10 ):_n(n){}static int plusi ( int a, int b){return a + b;}double plusd ( double a, double b){return (a + b) * _n;}private :int _n;};int main (){// 包装各种可调⽤对象function< int ( int , int )> f1 = f;function< int ( int , int )> f2 = Functor ();function< int ( int , int )> f3 = []( int a, int b) { return a + b; };cout << f1 ( 1 , 1 ) << endl;cout << f2 ( 1 , 1 ) << endl;cout << f3 ( 1 , 1 ) << endl;// 包装静态成员函数// 成员函数要指定类域并且前⾯加 & 才能获取地址function< int ( int , int )> f4 = &Plus::plusi;cout << f4 ( 1 , 1 ) << endl;// 包装普通成员函数// 普通成员函数还有⼀个隐含的 this 指针参数,所以绑定时传对象或者对象的指针过去都可以function< double (Plus*, double , double )> f5 = &Plus::plusd;Plus pd;cout << f5 (&pd, 1.1 , 1.1 ) << endl;function< double (Plus, double , double )> f6 = &Plus::plusd;cout << f6 (pd, 1.1 , 1.1 ) << endl;cout << f6 (pd, 1.1 , 1.1 ) << endl;function< double (Plus&&, double , double )> f7 = &Plus::plusd;cout << f7 ( move (pd), 1.1 , 1.1 ) << endl;cout << f7 ( Plus (), 1.1 , 1.1 ) << endl;return 0 ;}
8.2bind
simple ( 1 )template < class Fn , class ... Args>/* unspecified */ bind (Fn&& fn, Args&&... args);with return type ( 2 )template < class Ret, class Fn, class ... Args>/* unspecified */ bind (Fn&& fn, Args&&... args);
# include <functional>using placeholders::_1;using placeholders::_2;using placeholders::_3;int Sub ( int a, int b){return (a - b) * 10 ;}int SubX ( int a, int b, int c){return (a - b - c) * 10 ;}class Plus{public :static int plusi ( int a, int b){return a + b;}double plusd ( double a, double b){return a + b;}};int main (){auto sub1 = bind (Sub, _1, _2);cout << sub1 ( 10 , 5 ) << endl;// bind 本质返回的⼀个仿函数对象// 调整参数顺序(不常⽤)// _1 代表第⼀个实参// _2 代表第⼆个实参// ...auto sub2 = bind (Sub, _2, _1);cout << sub2 ( 10 , 5 ) << endl;// 调整参数个数 (常⽤)auto sub3 = bind (Sub, 100 , _1);cout << sub3 ( 5 ) << endl;auto sub4 = bind (Sub, _1, 100 );cout << sub4 ( 5 ) << endl;// 分别绑死第 123 个参数auto sub5 = bind (SubX, 100 , _1, _2);cout << sub5 ( 5 , 1 ) << endl;auto sub6 = bind (SubX, _1, 100 , _2);cout << sub6 ( 5 , 1 ) << endl;auto sub7 = bind (SubX, _1, _2, 100 );cout << sub7 ( 5 , 1 ) << endl;// 成员函数对象进⾏绑死,就不需要每次都传递了function< double (Plus&&, double , double )> f6 = &Plus::plusd;Plus pd;cout << f6 ( move (pd), 1.1 , 1.1 ) << endl;cout << f6 ( Plus (), 1.1 , 1.1 ) << endl;// bind ⼀般⽤于,绑死⼀些固定参数function< double ( double , double )> f7 = bind (&Plus::plusd, Plus (), _1, _2);cout << f7 ( 1.1 , 1.1 ) << endl;// 计算复利的 lambdaauto func1 = []( double rate, double money, int year)-> double {double ret = money;for ( int i = 0 ; i < year; i++){ret += ret * rate;}return ret - money;};// 绑死⼀些参数,实现出⽀持不同年华利率,不同⾦额和不同年份计算出复利的结算利息function< double ( double )> func3_1_5 = bind (func1, 0.015 , _1, 3 );function< double ( double )> func5_1_5 = bind (func1, 0.015 , _1, 5 );function< double ( double )> func10_2_5 = bind (func1, 0.025 , _1, 10 );function< double ( double )> func20_3_5 = bind (func1, 0.035 , _1, 30 );cout << func3_1_5 ( 1000000 ) << endl;cout << func5_1_5 ( 1000000 ) << endl;cout << func10_2_5 ( 1000000 ) << endl;cout << func20_3_5 ( 1000000 ) << endl;return 0 ;}
结束语C++11总结完毕,智能指针部分下篇博客总结OK,感谢观看!!!![]()